Nota
Haga clic aquí para descargar el código de ejemplo completo
Construyendo histogramas usando Rectangles y PolyCollections #
Usando un parche de ruta para dibujar rectángulos. La técnica de usar muchas instancias de Rectangle, o el método más rápido de usar PolyCollections, se implementaron antes de que tuviéramos rutas adecuadas con moveto/lineto, closepoly, etc. en mpl. Ahora que los tenemos, podemos dibujar colecciones de objetos de forma regular con propiedades homogéneas de manera más eficiente con PathCollection. Este ejemplo hace un histograma: es más trabajo configurar las matrices de vértices desde el principio, pero debería ser mucho más rápido para una gran cantidad de objetos.
import numpy as np
import matplotlib.pyplot as plt
import matplotlib.patches as patches
import matplotlib.path as path
fig, ax = plt.subplots()
# Fixing random state for reproducibility
np.random.seed(19680801)
# histogram our data with numpy
data = np.random.randn(1000)
n, bins = np.histogram(data, 50)
# get the corners of the rectangles for the histogram
left = bins[:-1]
right = bins[1:]
bottom = np.zeros(len(left))
top = bottom + n
# we need a (numrects x numsides x 2) numpy array for the path helper
# function to build a compound path
XY = np.array([[left, left, right, right], [bottom, top, top, bottom]]).T
# get the Path object
barpath = path.Path.make_compound_path_from_polys(XY)
# make a patch out of it
patch = patches.PathPatch(barpath)
ax.add_patch(patch)
# update the view limits
ax.set_xlim(left[0], right[-1])
ax.set_ylim(bottom.min(), top.max())
plt.show()
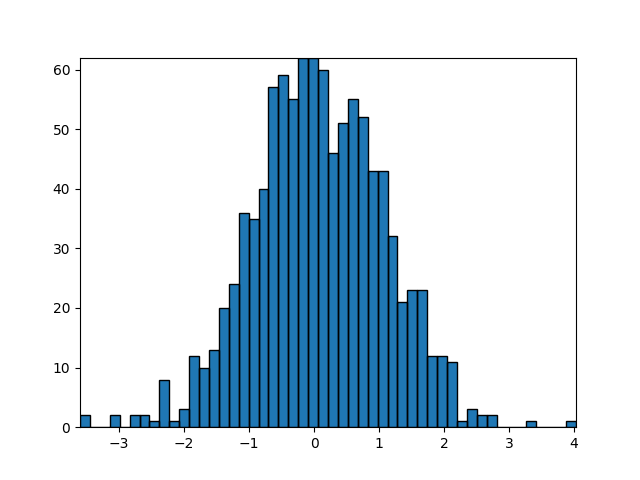
Cabe señalar que en lugar de crear una matriz tridimensional y usar make_compound_path_from_polys
, también podríamos crear la ruta compuesta directamente usando vértices y códigos como se muestra a continuación.
nrects = len(left)
nverts = nrects*(1+3+1)
verts = np.zeros((nverts, 2))
codes = np.ones(nverts, int) * path.Path.LINETO
codes[0::5] = path.Path.MOVETO
codes[4::5] = path.Path.CLOSEPOLY
verts[0::5, 0] = left
verts[0::5, 1] = bottom
verts[1::5, 0] = left
verts[1::5, 1] = top
verts[2::5, 0] = right
verts[2::5, 1] = top
verts[3::5, 0] = right
verts[3::5, 1] = bottom
barpath = path.Path(verts, codes)
Referencias
En este ejemplo se muestra el uso de las siguientes funciones, métodos, clases y módulos:
Este ejemplo muestra una alternativa a