Nota
Haga clic aquí para descargar el código de ejemplo completo
Estilos de caja personalizados #
Este ejemplo demuestra la implementación de un custom BoxStyle
. ConnectionStyle
Los s y s personalizados ArrowStyle
se pueden definir de manera similar.
from matplotlib.patches import BoxStyle
from matplotlib.path import Path
import matplotlib.pyplot as plt
Los estilos de cuadro personalizados se pueden implementar como una función que toma argumentos que especifican tanto un cuadro rectangular como la cantidad de "mutación", y devuelve la ruta "mutada". La firma específica es la de
custom_box_style
abajo.
Aquí, devolvemos una nueva ruta que agrega una forma de "flecha" a la izquierda del cuadro.
El estilo de cuadro personalizado se puede usar pasándolo
a .bbox=dict(boxstyle=custom_box_style, ...)
Axes.text
def custom_box_style(x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box around
it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Mutation reference scale, typically the text font size.
"""
# padding
mypad = 0.3
pad = mutation_size * mypad
# width and height with padding added.
width = width + 2 * pad
height = height + 2 * pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2), (x0, y0),
(x0, y0)],
closed=True)
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle=custom_box_style, alpha=0.2))
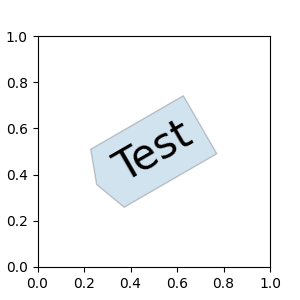
Text(0.5, 0.5, 'Test')
Del mismo modo, los estilos de cuadro personalizados se pueden implementar como clases que implementan
__call__
.
Luego, las clases se pueden registrar en el BoxStyle._style_list
dictado, lo que permite especificar el estilo del cuadro como una cadena,
. Tenga en cuenta que este registro se basa en API internas y, por lo tanto, no se admite oficialmente.bbox=dict(boxstyle="registered_name,param=value,...", ...)
class MyStyle:
"""A simple box."""
def __init__(self, pad=0.3):
"""
The arguments must be floats and have default values.
Parameters
----------
pad : float
amount of padding
"""
self.pad = pad
super().__init__()
def __call__(self, x0, y0, width, height, mutation_size):
"""
Given the location and size of the box, return the path of the box
around it.
Rotation is automatically taken care of.
Parameters
----------
x0, y0, width, height : float
Box location and size.
mutation_size : float
Reference scale for the mutation, typically the text font size.
"""
# padding
pad = mutation_size * self.pad
# width and height with padding added
width = width + 2.*pad
height = height + 2.*pad
# boundary of the padded box
x0, y0 = x0 - pad, y0 - pad
x1, y1 = x0 + width, y0 + height
# return the new path
return Path([(x0, y0),
(x1, y0), (x1, y1), (x0, y1),
(x0-pad, (y0+y1)/2.), (x0, y0),
(x0, y0)],
closed=True)
BoxStyle._style_list["angled"] = MyStyle # Register the custom style.
fig, ax = plt.subplots(figsize=(3, 3))
ax.text(0.5, 0.5, "Test", size=30, va="center", ha="center", rotation=30,
bbox=dict(boxstyle="angled,pad=0.5", alpha=0.2))
del BoxStyle._style_list["angled"] # Unregister it.
plt.show()
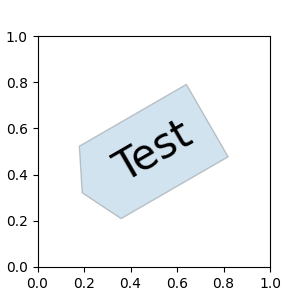