Nota
Haga clic aquí para descargar el código de ejemplo completo
Selectores de rectángulo y elipse #
Haga clic en algún lugar, mueva el mouse y suelte el botón del mouse.
RectangleSelector
y EllipseSelector
dibuje un rectángulo o una elipse desde la posición inicial del clic hasta la posición actual del mouse (dentro de los mismos ejes) hasta que se suelte el botón. Una devolución de llamada conectada recibe los eventos de clic y liberación.
from matplotlib.widgets import EllipseSelector, RectangleSelector
import numpy as np
import matplotlib.pyplot as plt
def select_callback(eclick, erelease):
"""
Callback for line selection.
*eclick* and *erelease* are the press and release events.
"""
x1, y1 = eclick.xdata, eclick.ydata
x2, y2 = erelease.xdata, erelease.ydata
print(f"({x1:3.2f}, {y1:3.2f}) --> ({x2:3.2f}, {y2:3.2f})")
print(f"The buttons you used were: {eclick.button} {erelease.button}")
def toggle_selector(event):
print('Key pressed.')
if event.key == 't':
for selector in selectors:
name = type(selector).__name__
if selector.active:
print(f'{name} deactivated.')
selector.set_active(False)
else:
print(f'{name} activated.')
selector.set_active(True)
fig = plt.figure(constrained_layout=True)
axs = fig.subplots(2)
N = 100000 # If N is large one can see improvement by using blitting.
x = np.linspace(0, 10, N)
selectors = []
for ax, selector_class in zip(axs, [RectangleSelector, EllipseSelector]):
ax.plot(x, np.sin(2*np.pi*x)) # plot something
ax.set_title(f"Click and drag to draw a {selector_class.__name__}.")
selectors.append(selector_class(
ax, select_callback,
useblit=True,
button=[1, 3], # disable middle button
minspanx=5, minspany=5,
spancoords='pixels',
interactive=True))
fig.canvas.mpl_connect('key_press_event', toggle_selector)
axs[0].set_title("Press 't' to toggle the selectors on and off.\n"
+ axs[0].get_title())
plt.show()
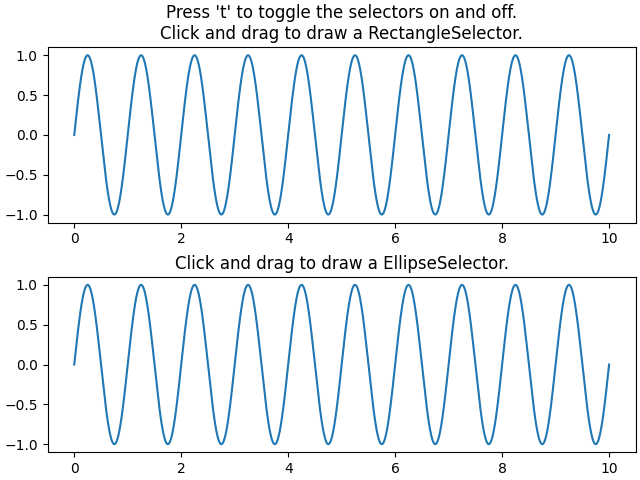
Referencias
En este ejemplo se muestra el uso de las siguientes funciones, métodos, clases y módulos: